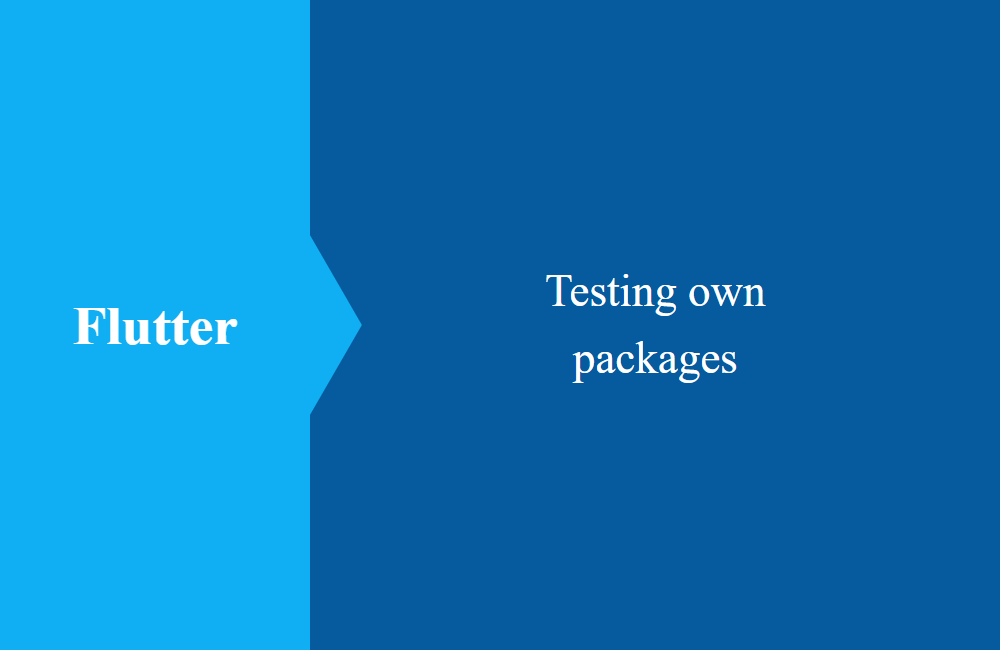
Flutter - Develop own packages (Part 2)
In the continuation of the series, we deal with the testing and further development of our own darts package from the last article.
Table of contents
In the first part, we mainly dealt with the implementation and use of the package. However, it is less clear how to actually develop the package further. That is why we want to refer to the topic of testing and further development today. If you haven't read the first part of the article, we recommend that you do so, so that you know what it's about.
Creation
When developing packages, it is considered best practice to also create an example for the other developers. This example is in the package in the example directory and represents a working prototype.
In the first step, we can run the built-in command "flutter create example" in our project, so that a new flutter app is created directly in our package. With this method, certain files are also not created, which we do not need in this example (CHANGELOG or LICENECE).
In the example directory you will find your usual structures and the file main.dart, in which you can do your example implementation. But before you can start with the implementation, you have to register your package via the pubspec.yaml file.
By specifying the path, we do not have to download the package first, but refer directly to the main package. So we only need some code in the main.dart.
import 'package:flutter/material.dart';
import 'package:testflutterpackage/pages/LoginPage.dart';
void main() {
runApp(TestApp());
}
class TestApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Test App',
home: LoginPage(),
);
}
}
Run
Normally you have to expand the configuration, otherwise you cannot start the sample app. To do this, create a new entry with the "+" symbol via the menu under "Run -> Edit Configurations ...". Choose Flutter, because we want to start a Flutter application. We assign main.dart as the name and store the path to the file, which serves as the entry point for the application for Dart.
Now you can start your device as usual and start with the main.dart for the test and expand the code. The hot reload works in the sample project, but also in the overall package that we are currently developing. One of the strengths of Flutter can be used as usual.
Conclusion
If you have components that need to be issued and you are developing the package further, it is definitely recommended to create an example. However, since this procedure is also common practice, you should also do it for other developers who may also use your package.