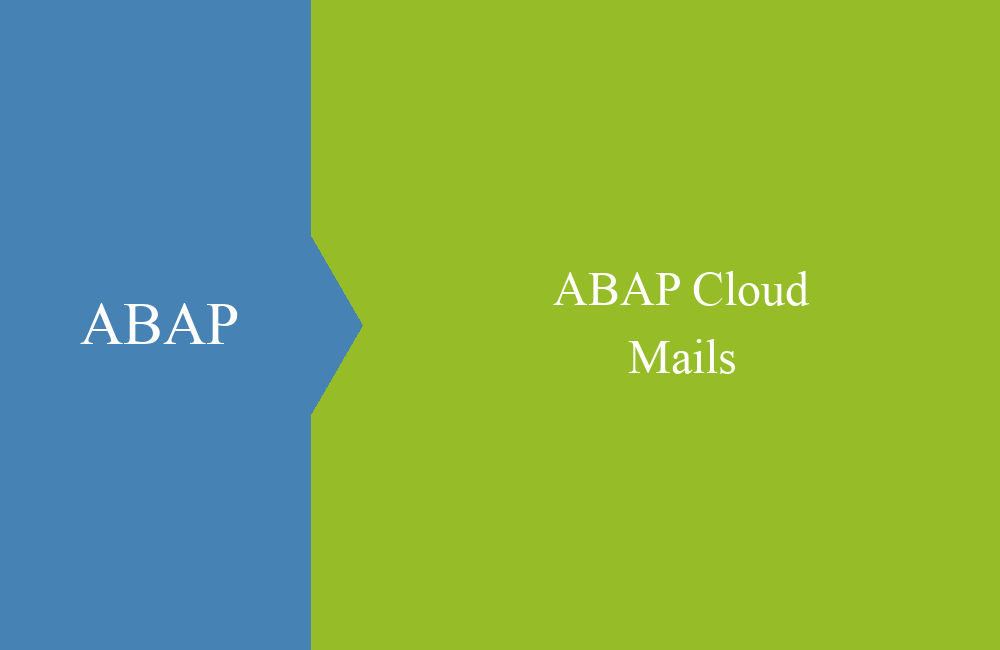
ABAP Cloud - Mails
Send emails with ABAP Cloud? Actually as easy as always, just with small differences that we want to clarify in this article.
Table of contents
In this article, you will learn a little more about the similarities and differences when creating and sending emails. We will look at the two worlds of on-premise and the ABAP environment. The two environments differ primarily in terms of configuration.
Introduction
Sending emails is used for different purposes in the system. Whether you want to send your colleague the latest financial report, receive a notification about the last ABAP unit test yourself, or simply be informed of errors. In many cases, classic email is used.
You can send these in the classic way as plain text or prepare them as an HTML document to distinguish yourself from your colleagues' emails. There are different classes available in the system for everything.
Comparison
In this section we will take a look at the classic world and the new world with ABAP Cloud.
Configuration
To configure the on-premise mail server, the appropriate setting can be made in the system and the mail server stored using the SCOT transaction.
A new configuration must be stored for the new send method. There are different configuration options depending on the system. You can find out how to create the mail connection in the ABAP environment in this article. To do this, the transaction SBCS_MAIL_CONFIGSMTP must be called on-premise. At the end, a default configuration should be found in the table BCS_MSG_SMTPCONF.
Creation
In classic ABAP, we mainly rely on the CL_BCS and CL_DOCUMENT_BCS classes to create our mail and then send it. First of all, we create a request, then we pass on the document and information about the sender and recipient. It is important that a COMMIT WORK follows at the end so that the email is passed on to the sending process.
DATA(lo_request) = cl_bcs=>create_persistent( ).
DATA(lt_text) = VALUE soli_tab(
( line = `<h2>Hello World</h2><p>This is my HTML Mail from <strong>Classic ABAP</strong>.</p>` ) ).
DATA(lo_document) = cl_document_bcs=>create_document( i_type = 'HTM'
i_subject = 'Test from Classic ABAP'
i_text = lt_text
i_importance = '5' ).
lo_request->set_document( lo_document ).
DATA(lo_address) = cl_cam_address_bcs=>create_internet_address( CONV #( c_sender ) ).
lo_request->set_sender( lo_address ).
DATA(lo_receiver1) = cl_cam_address_bcs=>create_internet_address( CONV #( c_receiver1 ) ).
lo_request->add_recipient( i_recipient = lo_receiver1
i_copy = abap_false ).
DATA(lo_receiver2) = cl_cam_address_bcs=>create_internet_address( CONV #( c_receiver2 ) ).
lo_request->add_recipient( i_recipient = lo_receiver2
i_copy = abap_true ).
COMMIT WORK.
In ABAP Cloud the main class for creating emails is CL_BCS_MAIL_MESSAGE, which has all the necessary functions for creating and sending. There is also the class CL_BCS_MAIL_BODYPART to create texts and attachments for the email. In addition to the Body class, there are also the two subclasses CL_BCS_MAIL_TEXTPART for pure text and CL_BCS_MAIL_BINARYPART for binary formats.
DATA(lo_mail) = cl_bcs_mail_message=>create_instance( ).
lo_mail->set_sender( c_sender ).
lo_mail->add_recipient( iv_address = c_receiver1
iv_copy = cl_bcs_mail_message=>to ).
lo_mail->add_recipient( iv_address = c_receiver2
iv_copy = cl_bcs_mail_message=>cc ).
lo_mail->set_subject( 'Test from ABAP Cloud' ).
DATA(ld_content) = `<h2>Hello World</h2><p>This is my HTML Mail from <strong>ABAP Cloud</strong>.</p>`.
lo_mail->set_main( cl_bcs_mail_textpart=>create_instance( iv_content = ld_content
iv_content_type = 'text/html' ) ).
lo_mail->send( IMPORTING et_status = DATA(lt_status)
ev_mail_status = DATA(ld_mail_status) ).
In the example, we create the instance of the Mail class, set the sender, the recipients and the texts and send the message. As a result, we get a status for each recipient as well as an overall status of the email.
Monitoring
In the SAP GUI there is the transaction SOST, SOSG or SOSB to view the send requests and what the current status is. In the transaction you can also filter according to different settings to get the desired result.
All emails sent via the new Cloud API can only be viewed via the corresponding Fiori app. A corresponding message also appears when you open the SOST and the Cloud scenario is activated. The Fiori app is "Monitor Email Transmissions" (F5442).
Complete example
Here is the complete example of sending emails from the system, once via the classic route and once via ABAP Cloud. The corresponding configurations must already have been carried out for the sending to work.
CLASS zcl_bs_demo_mail DEFINITION
PUBLIC FINAL
CREATE PUBLIC.
PUBLIC SECTION.
INTERFACES if_oo_adt_classrun.
PRIVATE SECTION.
CONSTANTS c_sender TYPE cl_bcs_mail_message=>ty_address VALUE '<MAIL>'.
CONSTANTS c_receiver1 TYPE cl_bcs_mail_message=>ty_address VALUE '<MAIL>'.
CONSTANTS c_receiver2 TYPE cl_bcs_mail_message=>ty_address VALUE '<MAIL>'.
METHODS classic_mail
IMPORTING io_out TYPE REF TO if_oo_adt_classrun_out.
METHODS cloud_mail
IMPORTING io_out TYPE REF TO if_oo_adt_classrun_out.
ENDCLASS.
CLASS zcl_bs_demo_mail IMPLEMENTATION.
METHOD if_oo_adt_classrun~main.
classic_mail( out ).
cloud_mail( out ).
ENDMETHOD.
METHOD classic_mail.
DATA(lo_request) = cl_bcs=>create_persistent( ).
DATA(lt_text) = VALUE soli_tab(
( line = `<h2>Hello World</h2><p>This is my HTML Mail from <strong>Classic ABAP</strong>.</p>` ) ).
DATA(lo_document) = cl_document_bcs=>create_document( i_type = 'HTM'
i_subject = 'Test from Classic ABAP'
i_text = lt_text
i_importance = '5' ).
lo_request->set_document( lo_document ).
DATA(lo_address) = cl_cam_address_bcs=>create_internet_address( CONV #( c_sender ) ).
lo_request->set_sender( lo_address ).
DATA(lo_receiver1) = cl_cam_address_bcs=>create_internet_address( CONV #( c_receiver1 ) ).
lo_request->add_recipient( i_recipient = lo_receiver1
i_copy = abap_false ).
DATA(lo_receiver2) = cl_cam_address_bcs=>create_internet_address( CONV #( c_receiver2 ) ).
lo_request->add_recipient( i_recipient = lo_receiver2
i_copy = abap_true ).
io_out->write( lo_request->send( ) ).
COMMIT WORK.
ENDMETHOD.
METHOD cloud_mail.
DATA(lo_mail) = cl_bcs_mail_message=>create_instance( ).
lo_mail->set_sender( c_sender ).
lo_mail->add_recipient( iv_address = c_receiver1
iv_copy = cl_bcs_mail_message=>to ).
lo_mail->add_recipient( iv_address = c_receiver2
iv_copy = cl_bcs_mail_message=>cc ).
lo_mail->set_subject( 'Test from ABAP Cloud' ).
DATA(ld_content) = `<h2>Hello World</h2><p>This is my HTML Mail from <strong>ABAP Cloud</strong>.</p>`.
lo_mail->set_main( cl_bcs_mail_textpart=>create_instance( iv_content = ld_content
iv_content_type = 'text/html' ) ).
lo_mail->send( IMPORTING et_status = DATA(lt_status)
ev_mail_status = DATA(ld_mail_status) ).
io_out->write( lt_status ).
io_out->write( |Mail status: { ld_mail_status }| ).
ENDMETHOD.
ENDCLASS.
At the end we receive a formatted email in HTML format. There are largely no limits to how you can format the email.
Availability
The email classes are available from S/4 HANA 2022 and can be used as described. However, the asynchronous sending of emails (SEND_ASYNC method) will only be available with S/4 HANA 2023, as the method relies on the bgPF framework.
Conclusion
With ABAP Cloud, there are also some changes in the system and configuration when sending emails. There will probably be little change in the preparation of documents, but there will be changes in how documents are sent.