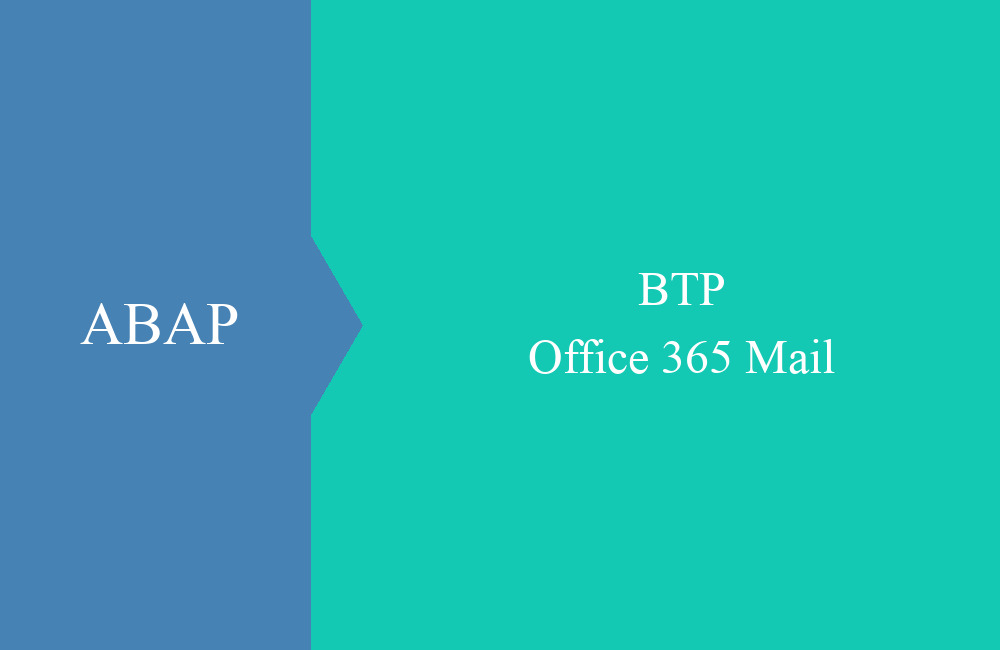
BTP - Connect Office365 Mail
In this article we want to look at how we connect and configure an Office365 mail server to the ABAP environment.
Table of contents
The ABAP environment does not usually have its own mail server and therefore needs a configuration to be able to send e-mails. In this article we will go into the connection of a cloud SMTP server and how we connect it to the ABAP environment to send e-mails.
Introduction
We use the Office365 server for the connection, which most companies use to process their e-mails if they rely on Microsoft products. We work with a technical user and the basic authentication (user and password) to log on to the server.
Connection
To set up the connection, we need to call the "Communication Systems" app in the launchpad of the ABAP Environment. There we create a new system via "New". The system ID and the name are irrelevant, so they can be freely assigned.
Next, in the "Technical Data" section, we enter the server and port for Office365, you can easily find the data using the search engine of your choice:
The authentication must now be created in the "Users for Outbound Communication" section. To do this, we choose the User/Password method to log into the server.
For the last step we have to switch to the "Communiation Arrangements" app in order to connect the scenario to the system there. We create a corresponding arrangement via the "New" button. You can find the scenario using the search help if you search for "Mail". We need the ID SAP_COM_0548:
Now, in the "Common Data" section, only the scenario needs to be connected to the communication system, which completes the basic configuration in the system:
Configuration
After the connection to the server has been established, we still have to configure the system. This includes maintaining the sender and recipient lists, without this configuration no mail can be sent from the system. The API is currently only available in the form of ABAP classes and not as a Fiori app, so further configuration is done via an executable ABAP class.
Set
In the first step we set the most important settings, for this we need a mail configuration object in the first step, which we create via the corresponding API:
DATA(lo_config) = cl_bcs_mail_system_config=>create_instance( ).
We can now use this object to set the other settings, activate the check, set the expiry date and set the default sender:
lo_config->set_address_check_active( abap_true ).
lo_config->set_days_until_mail_expires( 7 ).
lo_config->modify_default_sender_address( iv_default_address = 'BTP-noreply@CONNECT.com'
iv_default_name = '' ).
If the address check is now activated, we have to set the sender and recipient in the next step so that we can set the e-mails from the system.
" Set sender
DATA(lt_allowed_sender) = VALUE cl_bcs_mail_system_config=>tyt_sender_domains( ( '*@CONNECT.com' ) ).
lo_config->add_allowed_sender_domains( it_allowed_sender_domains = lt_allowed_sender ).
" Set receiver
DATA(lt_allowed_receiver) = VALUE cl_bcs_mail_system_config=>tyt_recipient_domains( ( '*@CONNECT.com' )
( '*@OTHERS.com' ) ).
lo_config->add_allowed_recipient_domains( it_allowed_rec_domains = lt_allowed_receiver ).
Hint: We can work with wildcards to activate the domains. Since in our case the sender is the default sender, one domain is sufficient. All relevant domains should be activated as recipients.
Get
You can also read the set configuration accordingly in order to check the settings and adjust them again later. With the API you have the possibility to also provide an application for maintenance. For example code for reading the data, see the full example below.
Full example
As you are already used to from our old articles, here is the complete example coding in the form of the executable class. The methods in the main method can be commented in and out accordingly.
CLASS zcl_bs_demo_mail_config DEFINITION
PUBLIC
FINAL
CREATE PUBLIC.
PUBLIC SECTION.
INTERFACES if_oo_adt_classrun.
PRIVATE SECTION.
METHODS get_configuration
IMPORTING io_out TYPE REF TO if_oo_adt_classrun_out.
METHODS set_configuration
IMPORTING io_out TYPE REF TO if_oo_adt_classrun_out.
ENDCLASS.
CLASS zcl_bs_demo_mail_config IMPLEMENTATION.
METHOD if_oo_adt_classrun~main.
get_configuration( out ).
* set_configuration( out ).
ENDMETHOD.
METHOD get_configuration.
DATA(lo_config) = cl_bcs_mail_system_config=>create_instance( ).
io_out->write( |Check active: { lo_config->get_address_check_active( ) }| ).
io_out->write( |Expires: { lo_config->get_days_until_mail_expires( ) }| ).
io_out->write( |Sender domains:| ).
DATA(lt_sender_domains) = lo_config->read_allowed_sender_domains( ).
io_out->write( lt_sender_domains ).
io_out->write( |Receiver domains:| ).
DATA(lt_rec_domains) = lo_config->read_allowed_recipient_domains( ).
io_out->write( lt_rec_domains ).
lo_config->read_default_sender_address( IMPORTING ev_default_sender_address = DATA(ld_address)
ev_default_sender_name = DATA(ld_name) ).
io_out->write( |Sender address: { ld_address }| ).
io_out->write( |Sender name: { ld_name }| ).
ENDMETHOD.
METHOD set_configuration.
DATA(lo_config) = cl_bcs_mail_system_config=>create_instance( ).
TRY.
lo_config->set_address_check_active( abap_true ).
io_out->write( `Check activated` ).
CATCH cx_bcs_mail_config.
io_out->write( `Error in activation` ).
RETURN.
ENDTRY.
TRY.
lo_config->set_days_until_mail_expires( 7 ).
io_out->write( `Expiry days set to 7` ).
CATCH cx_bcs_mail_config.
io_out->write( `Error while set the new days` ).
ENDTRY.
TRY.
DATA(lt_allowed_receiver) = VALUE cl_bcs_mail_system_config=>tyt_recipient_domains( ( '*@CONNECT.com' )
( '*@OTHERS.com' ) ).
lo_config->add_allowed_recipient_domains( it_allowed_rec_domains = lt_allowed_receiver ).
io_out->write( `Allowlist receiver added` ).
CATCH cx_bcs_mail_config.
io_out->write( `Error in allowlist receiver` ).
ENDTRY.
TRY.
DATA(lt_allowed_sender) = VALUE cl_bcs_mail_system_config=>tyt_sender_domains( ( '*@CONNECT.com' ) ).
lo_config->add_allowed_sender_domains( it_allowed_sender_domains = lt_allowed_sender ).
io_out->write( `Allowlist sender added` ).
CATCH cx_bcs_mail_config.
io_out->write( `Error in allowlist sender` ).
ENDTRY.
TRY.
lo_config->modify_default_sender_address( iv_default_address = 'BTP-noreply@CONNECT.com'
iv_default_name = '' ).
io_out->write( `Default sender set` ).
CATCH cx_bcs_mail_config.
io_out->write( `Error with default data` ).
ENDTRY.
ENDMETHOD.
ENDCLASS.
Conclusion
The basic configuration of the mail server and the system is not that complicated, but requires some steps of preparation and some data to finalize the configuration. You should also have the necessary apps and permissions when configuring the system.