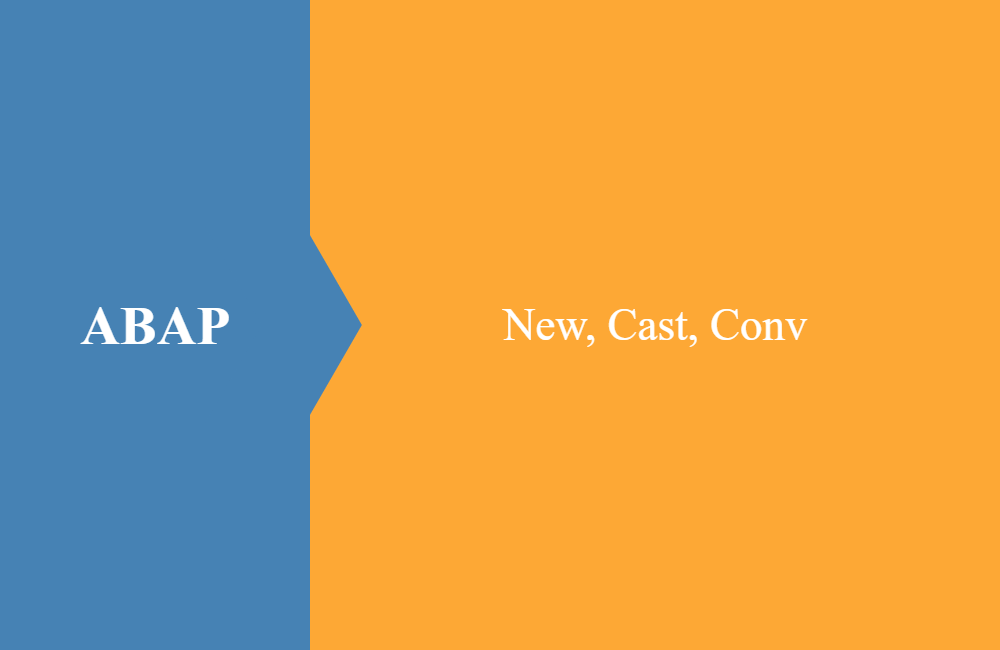
ABAP - New, Cast, Conv
Many of the new expressions are so-called constructor expressions. What exactly that means for you and what it brings you find out here in our article.
Table of contents
Constructor expressions or inline functions are functions that can be used in a line of code and also return a result, value or object. They are the opposite of the one-line expressions from the Cobol times, which are still very much in the system.
Take as an example a simple data assignment. This was done by Move and was very easy to read for everyone. With the direct assignment with "=" you can now express this in a single line, saves a lot of typing and is just as easy to read:
DATA:
ld_one TYPE c LENGTH 10,
ld_two TYPE c LENGTH 10.
" old assignment
MOVE 'COUNT' TO ld_one.
MOVE ld_one TO ld_two.
" new assignment
ld_two = ld_one = 'COUNT'.
Inline functions can now be nested as desired, of course only where it makes sense, and can be used very flexibly. The first three expressions we want to introduce you today.
New
The command creates an object of a class and replaces the old "Create Object". The type of the class is implicitly derived from the data type of the target variable, or it is explicitly specified as the usage type. Of course, the second form of the definition saves one line and shows the advantage of the linked functions.
" implicit generation
DATA lo_alv TYPE REF TO cl_gui_alv_grid.
lo_alv = NEW #( ... ).
" explicit generation
DATA(lo_alv) = NEW cl_gui_alv_grid( ... ).
Cast
The cast function is used for up/downcasting objects. Previously, it was done with a simple assignment over "=" or the assignment "? =". However, this form of assignment was not always clear and somewhat confusing for some developers. For this purpose they have been implemented this function, which can always be used and shows what happens at this point of the coding. Again, both forms of generation in comparison:
DATA:
lo_dock TYPE REF TO cl_gui_docking_container,
lo_cont TYPE REF TO cl_gui_container.
" implicit generation
lo_cont = CAST #( lo_dock ).
" explicit generation
DATA(lo_cont) = CAST cl_gui_container( lo_dock ).
In the second case, you do not need the previous definition of lo_cont because you create it at runtime. The Cast command sees the statement at first glance and the developer knows that the type of object has been adjusted here.
Conv
The new statement converts a source value into a new target value with a new type and, like all other new expressions, it can also be used concatenated.
Most useful is the function of supplying parameters when they demand a stricter type. Then you can map the variable to the appropriate target type and save yourself the investment of an intermediate variable and the filling of this.
" Conversion 12 -> 0012
DATA(ld_num) = CONV numc4( 12 ).
" Usage as parameter
DATA:
ld_name TYPE string VALUE 'ZTEST_JOBNAME',
lt_log TYPE STANDARD TABLE OF tbtc5.
CALL FUNCTION 'BP_JOBLOG_READ'
EXPORTING
jobname = CONV btcjob( ld_name )
TABLES
joblogtbl = lt_log.
In the second example, we read a joblog from the system but only have the job name in a string. However, a call with this parameter would cause the system to dump because the parameter has a different data type. However, with the conversion to the correct data type, we save ourselves the creation of an intermediate variable.
Conclusion
With the first new features you get a lot of little helpers on hand, which support your developer life in many ways and make it faster. The direct generation of new variables at runtime also saves a lot of code and makes your coding much clearer.
Source:
SAP Documentation New
SAP Documentation Cast
SAP Documentation Conv